9. COMPUTER GRAPHICS LAB | READ NOW
VTU COMPUTER GRAPHICS LAB
Program 9:- Develop a menu driven program to fill the polygon using scan line algorithm
STEPS TO RUN CG PROGRAM
- Copy the below copy
- Past it in any code compiler ex- Code Blocks, DEV C++, VS Code
- Save the file with .cpp extension
- Compile and Run the code
- Program Execution Successful
Note:- if you use Dev C++, right click on project>project options>parameter>linker paste the below code in Linker – as shown in image.
-lopengl32 -lfreeglut -lglu32
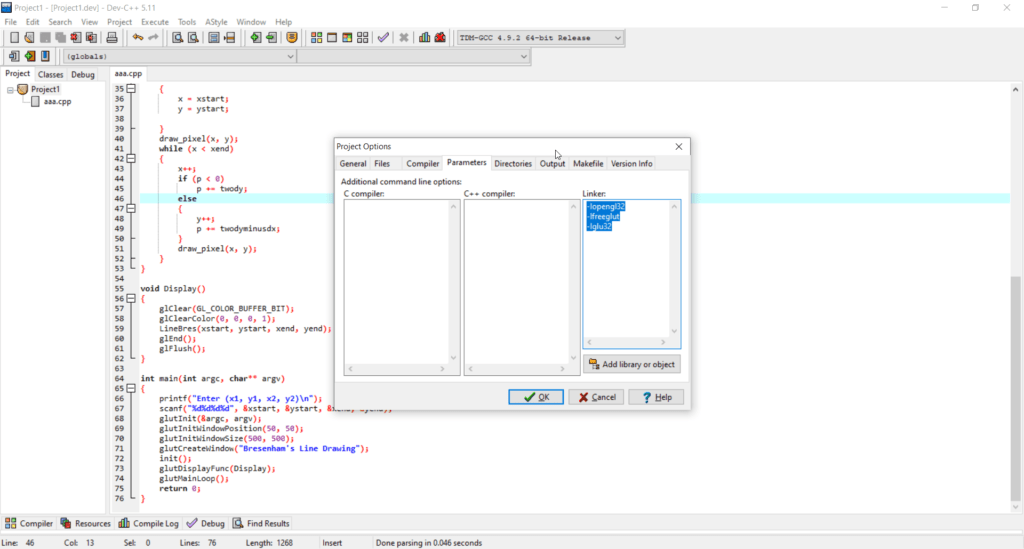
Program Code [lab9.cpp]
#include <stdlib.h> #include <stdio.h> #include <GL/glut.h> float x1,x2,x3,x4,y1,y2,y3,y4; void edgedetect(float x1,float y1,float x2,float y2,int *le,int *re) { float mx,x,temp; int i; if((y2-y1)<0) { temp=y1;y1=y2;y2=temp; temp=x1;x1=x2;x2=temp; } if((y2-y1)!=0) mx=(x2-x1)/(y2-y1); else mx=x2-x1; x=x1; for(i=y1;i<=y2;i++) { if(x<(float)le[i]) le[i]=(int)x; if(x>(float)re[i]) re[i]=(int)x; x+=mx; } } void draw_pixel(int x,int y) { glColor3f(1.0,1.0,0.0); glBegin(GL_POINTS); glVertex2i(x,y); glEnd(); } void scanfill(float x1,float y1,float x2,float y2,float x3,float y3,float x4,float y4) { int le[500],re[500]; int i,y; for(i=0;i<500;i++) { le[i]=500; re[i]=0; } edgedetect(x1,y1,x2,y2,le,re); edgedetect(x2,y2,x3,y3,le,re); edgedetect(x3,y3,x4,y4,le,re); edgedetect(x4,y4,x1,y1,le,re); for(y=0;y<500;y++) { if(le[y]<=re[y]) for(i=(int)le[y];i<(int)re[y];i++) draw_pixel(i,y); } } void display() { x1=200.0;y1=200.0;x2=100.0;y2=300.0;x3=200.0;y3=400.0;x4=300.0;y4=300.0; glClear(GL_COLOR_BUFFER_BIT); glColor3f(0.0, 0.0, 1.0); glBegin(GL_LINE_LOOP); glVertex2f(x1,y1); glVertex2f(x2,y2); glVertex2f(x3,y3); glVertex2f(x4,y4); glEnd(); scanfill(x1,y1,x2,y2,x3,y3,x4,y4); glFlush(); } void myinit() { glClearColor(1.0,1.0,1.0,1.0); glColor3f(1.0,0.0,0.0); glPointSize(1.0); glMatrixMode(GL_PROJECTION); glLoadIdentity(); gluOrtho2D(0.0,499.0,0.0,499.0); } int main(int argc, char** argv) { glutInit(&argc,argv); glutInitDisplayMode(GLUT_SINGLE|GLUT_RGB); glutInitWindowSize(500,500); glutInitWindowPosition(0,0); glutCreateWindow("Filling a Polygon using Scan-line Algorithm"); glutDisplayFunc(display); myinit(); glutMainLoop(); }
COMPUTER GRAPHICS OUTPUT

Alternative Method [lab9.cpp]
#include<stdio.h> #include<math.h> #include<iostream> #include<GL/glut.h> int le[500], re[500], flag=0 ,m; void init() { gluOrtho2D(0, 500, 0, 500); } void edge(int x0, int y0, int x1, int y1) { if (y1<y0) { int tmp; tmp = y1; y1 = y0; y0 = tmp; tmp = x1; x1 = x0; x0 = tmp; } int x = x0; m = (y1 - y0) / (x1 - x0); for (int i = y0; i<y1; i++) { if (x<le[i]) le[i] = x; if (x>re[i]) re[i] = x; x += (1 / m); } } void display() { glClearColor(1, 1, 1, 1); glClear(GL_COLOR_BUFFER_BIT); glColor3f(0, 0, 1); glBegin(GL_LINE_LOOP); glVertex2f(200, 100); glVertex2f(100, 200); glVertex2f(200, 300); glVertex2f(300, 200); glEnd(); for (int i = 0; i<500; i++) { le[i] = 500; re[i] = 0; } edge(200, 100, 100, 200); edge(100, 200, 200, 300); edge(200, 300, 300, 200); edge(300, 200, 200, 100); if (flag == 1) { for (int i = 0; i < 500; i++) { if (le[i] < re[i]) { for (int j = le[i]; j < re[i]; j++) { glColor3f(1, 0, 0); glBegin(GL_POINTS); glVertex2f(j, i); glEnd(); } } } } glFlush(); } void ScanMenu(int id) { if (id == 1) { flag = 1; } else if (id == 2) { flag = 0; } else { exit(0); } glutPostRedisplay(); } int main(int argc, char **argv) { glutInit(&argc, argv); glutInitWindowPosition(100, 100); glutInitWindowSize(500, 500); glutCreateWindow("scan line"); init(); glutDisplayFunc(display); glutCreateMenu(ScanMenu); glutAddMenuEntry("scanfill", 1); glutAddMenuEntry("clear", 2); glutAddMenuEntry("exit", 3); glutAttachMenu(GLUT_RIGHT_BUTTON); glutMainLoop(); return 0; }
COMPUTER GRAPHICS Alternative method output
