4. COMPUTER GRAPHICS LAB | READ NOW
VTU COMPUTER GRAPHICS LAB
Program 4:- Draw a color cube and allow the user to move the camera suitably to experiment with
perspective viewing
STEPS TO RUN CG PROGRAM
- Copy the below copy
- Past it in any code compiler ex- Code Blocks, DEV C++, VS Code
- Save the file with .cpp extension
- Compile and Run the code
- Program Execution Successful
Note:- if you use Dev C++, right click on project>project options>parameter>linker paste the below code in Linker – as shown in image.
-lopengl32 -lfreeglut -lglu32
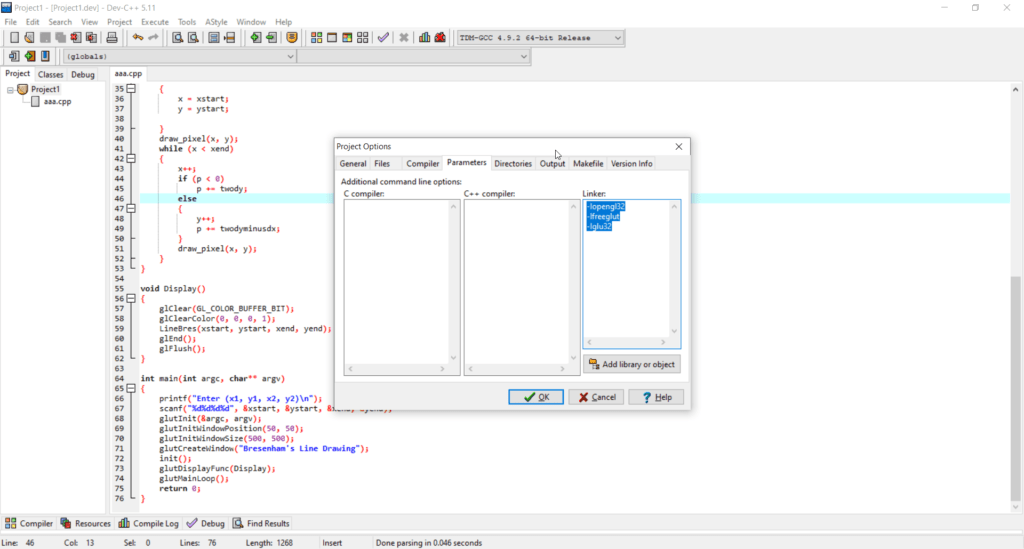
Program Code [lab4.cpp]
#include <stdlib.h> #include <GL/glut.h> #include <stdio.h> GLfloat vertices[ ]={ -1.0,-1.0,-1.0, 1.0,-1.0,-1.0, 1.0, 1.0,-1.0, -1.0, 1.0,-1.0, -1.0,-1.0, 1.0, 1.0,-1.0, 1.0, 1.0, 1.0, 1.0, -1.0, 1.0, 1.0 }; GLfloat normals[ ] ={ -1.0,-1.0,-1.0, 1.0,-1.0,-1.0, 1.0, 1.0,-1.0, -1.0, 1.0,-1.0, -1.0,-1.0, 1.0, 1.0,-1.0, 1.0, 1.0, 1.0, 1.0, -1.0, 1.0, 1.0 }; GLfloat colors[ ]={ 0.0, 0.0, 0.0, 1.0, 0.0, 0.0, 1.0, 1.0, 0.0, 0.0, 1.0, 0.0, 0.0, 0.0, 1.0, 1.0, 0.0, 1.0, 1.0, 1.0, 1.0, 0.0, 1.0, 1.0}; GLubyte cubeIndices[]={ 0,3,2,1, 2,3,7,6, 0,4,7,3, 1,2,6,5, 4,5,6,7, 0,1,5,4}; static GLfloat theta[] = {0.0,0.0,0.0}; static GLint axis = 2; static GLdouble viewer[] = {0.0,0.0,5.0}; void display(void) { glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glLoadIdentity(); gluLookAt(viewer[0],viewer[1],viewer[2],0.0,0.0,0.0,0.0,1.0,0.0); glRotatef(theta[0],1.0,0.0,0.0); glRotatef(theta[1],0.0,1.0,0.0); glRotatef(theta[2],0.0,0.0,1.0); glDrawElements(GL_QUADS,24,GL_UNSIGNED_BYTE,cubeIndices); glFlush(); glutSwapBuffers(); } void mouse(int btn, int state, int x, int y) { if(btn==GLUT_LEFT_BUTTON && state==GLUT_DOWN) axis=0; if(btn==GLUT_RIGHT_BUTTON && state==GLUT_DOWN) axis=1; if(btn==GLUT_MIDDLE_BUTTON && state==GLUT_DOWN) axis=2; theta[axis]+=2.0; if(theta[axis]>360.0) theta[axis]-=360.0; glutPostRedisplay(); } void keys(unsigned char key, int x, int y) { if(key=='x') viewer[0]-=1.0; if(key=='X') viewer[0]+=1.0; if(key=='y') viewer[1]-=1.0; if(key=='Y') viewer[1]+=1.0; if(key=='z') viewer[2]-=1.0; if(key=='Z') viewer[2]+=1.0; glutPostRedisplay(); } void myReshape(int w, int h) { glViewport(0,0,w,h); glMatrixMode(GL_PROJECTION); glLoadIdentity(); if(w<=h) glFrustum(-2.0,2.0,-2.0*(GLfloat)h/(GLfloat)w,2.0*(GLfloat)h/(GLfloat)w,2.0,20.0); else glFrustum(-2.0,2.0,-2.0*(GLfloat)w/(GLfloat)h,2.0*(GLfloat)w/(GLfloat)h,2.0,20.0); glMatrixMode(GL_MODELVIEW); } int main(int argc, char **argv) { glutInit(&argc,argv); glutInitDisplayMode(GLUT_DOUBLE|GLUT_RGB|GLUT_DEPTH); glutInitWindowSize(500,500); glutCreateWindow("color cuce"); glutReshapeFunc(myReshape); glutDisplayFunc(display); glutKeyboardFunc(keys); glutMouseFunc(mouse); glEnable(GL_DEPTH_TEST); glEnableClientState(GL_COLOR_ARRAY); glEnableClientState(GL_VERTEX_ARRAY); glEnableClientState(GL_NORMAL_ARRAY); glVertexPointer(3,GL_FLOAT,0,vertices); glColorPointer(3,GL_FLOAT,0,colors); glNormalPointer(GL_FLOAT,0,normals); glColor3f(1.0,1.0,1.0); glutMainLoop(); }
COMPUTER GRAPHICS LAB4 OUTPUT

Alternative Method [lab4.cpp]
#include<stdio.h> #include<math.h> #include<iostream> #include<GL/glut.h> using namespace std; float pts[8][3] = {{-1,-1,-1},{-1,1,-1},{1,1,-1},{1,-1,-1},{-1,-1,1},{-1,1,1},{1,1,1},{1,-1,1}}; float theta[] ={0,0,0}; int axis = 2; float viewer[]={5,0,0}; void myInit() { glMatrixMode(GL_PROJECTION); glFrustum(-2,2,-2,2,2,10); glMatrixMode(GL_MODELVIEW); } void draw_polygon(int a, int b, int c, int d) { glBegin(GL_QUADS); glVertex3fv(pts[a]); glVertex3fv(pts[b]); glVertex3fv(pts[c]); glVertex3fv(pts[d]); glEnd(); } void draw_cube(float pts[8][3]) { glColor3f(0,0,1); draw_polygon(0,1,2,3); //front face glColor3f(0,1,0); draw_polygon(4,5,6,7); //behind face glColor3f(1,0,0); draw_polygon(0,1,5,4); //left face glColor3f(0,0,0); draw_polygon(3,2,6,7); //right face glColor3f(0,1,1); draw_polygon(0,4,7,3); //bottom face glColor3f(1,0,1); draw_polygon(1,5,6,2); //top face } void myDisplay() { glClearColor(1,1,1,1); glClear(GL_COLOR_BUFFER_BIT|GL_DEPTH_BUFFER_BIT); glLoadIdentity(); gluLookAt(viewer[0],viewer[1],viewer[2],0,0,0,0,1,0); glRotatef(theta[2],0,0,1); glRotatef(theta[1],0,1,0); glRotatef(theta[0],1,0,0); draw_cube(pts); glFlush(); glutSwapBuffers(); } void spincube() { theta[axis] = theta[axis]+4; if(theta[axis]>360) theta[axis]=0; glutPostRedisplay(); } void mouse(int btn , int state , int x , int y) { if((btn==GLUT_LEFT_BUTTON)&&(state==GLUT_DOWN)) axis=0; if((btn==GLUT_RIGHT_BUTTON)&&(state==GLUT_DOWN)) axis=2; if((btn==GLUT_MIDDLE_BUTTON)&&(state==GLUT_DOWN)) axis=1; spincube(); } void keyboard(unsigned char key, int x, int y) { if(key=='X') viewer[0]+=1; if(key=='x') viewer[0]-=1; if(key=='Y') viewer[1]+=1; if(key=='y') viewer[1]-=1; if(key=='Z') viewer[2]+=1; if(key=='z') viewer[2]-=1; glutPostRedisplay(); } int main (int argc, char ** argv) { glutInit(&argc,argv); glutInitDisplayMode( GLUT_DOUBLE|GLUT_RGB|GLUT_DEPTH); glutInitWindowPosition(50,50); glutInitWindowSize(500,500); glutCreateWindow("Positioning of Camera"); myInit(); glEnable(GL_DEPTH_TEST); glutDisplayFunc(myDisplay); glutKeyboardFunc(keyboard); glutMouseFunc(mouse); glutMainLoop(); }
Alternative COMPUTER GRAPHICS LAB4 Output
