Design and Analysis of Algorithm Lab 2 | Read Now
Design and Analysis of Algorithm Lab 2
2A] Design a superclass called staff with details as StaffId, Name, Phone, Salary. Extend this class by writing three sub-classes namely Teaching(domain, publications), Technical(skills), and Contract(period). Write a Java Program to read and display at least 3 staff objects of all the 3 categories.
2B] Write a Java class called the customer to store their name and date_of_birth. The date_of_birth format should be dd/mm/yyyy. Write methods to read customer data as <name, dd/mm/yyyy> and display as <name, dd, mm, yyyy> using StringTokenizer class considering the delimiter character as ” / “.
2A] – Program code
class Staff { private int StaffId; private String Name; private String Phone; private long Salary; public Staff(int staffId,String name,String phone,long salary) { StaffId = staffId; Name = name; Phone = phone; Salary = salary; } public void Display() { System.out.print("\t"+StaffId+"\t"+Name+"\t\t"+Phone+"\t\t"+Salary); } } class Teaching extends Staff { private String Domain; private int Publications; public Teaching(int staffId, String name, String phone,long salary, String domain, int publications) { super(staffId, name, phone, salary); Domain = domain; Publications = publications; } public void Display() { super.Display(); System.out.print("\t\t"+Domain+"\t\t"+Publications+"\t\t"+"--"+"\t"+"--"); } } class Technical extends Staff { private String Skills; public Technical(int staffId, String name, String phone,long salary, String skills) { super(staffId, name, phone, salary); Skills = skills; } public void Display() { super.Display(); System.out.print("\t\t--"+"\t\t"+"--"+"\t"+Skills+"\t"+"--"); } } class Contract extends Staff { private int Period; public Contract(int staffId, String name, String phone, long salary, int period) { super(staffId, name, phone, salary); this.Period = period; } public void Display() { super.Display(); System.out.print("\t\t--"+"\t\t"+"--"+"\t\t"+"--"+"\t"+Period); } } public class lab2a { public static void main(String[] args) { Staff staff[]=new Staff[3]; staff[0]=new Teaching(0001,"Narendr","271173",90000,"CSE",3); staff[1]=new Technical(0002,"Ara","271172",2000,"Server Admin"); staff[2]=new Contract(0003,"Rahul","271174",9000,3); System.out.println("Staff ID\tName\t\tPhone\t\tSalary\t\tDomain\tPublication\tSkills\t\tPeriod"); for(int i=0;i<3;i++) { staff[i].Display(); System.out.println(); } } }
Output

2B] Program code
import java.util.Scanner; import java.util.StringTokenizer; class Customer { private String name; private String date_of_birth; public Customer(String name, String date_of_birth) { super(); this.name = name; this.date_of_birth = date_of_birth; } public Customer () { } public void readData(String name, String date_of_birth) { this.name = name; this.date_of_birth = date_of_birth; } public void displayData() { StringTokenizer st=new StringTokenizer(this.date_of_birth,"/"); System.out.print(this.name+","); while(st.hasMoreTokens()) { System.out.print(st.nextToken()+","); } } } public class lab2b { public static void main(String[] args) { Scanner in=new Scanner(System.in); System.out.println("Enter Name :-"); String name=in.nextLine(); System.out.println("Enter Date of birth:-"); String date=in.next(); Customer customer=new Customer(); customer.readData(name, date); customer.displayData(); } }
Output
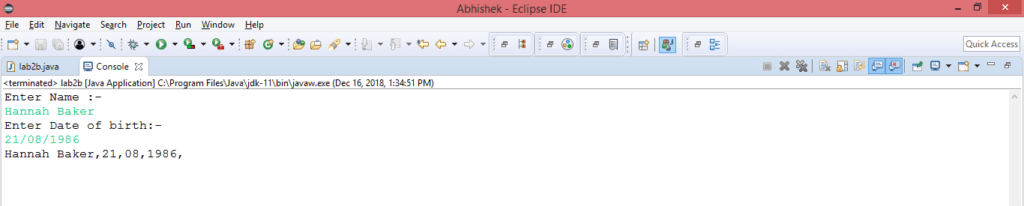